In this detailed guide, we will create a C# console application to connect to a Dynamics 365 CRM instance, identify duplicate contact records based on specific criteria, merge them into a single record, and export the merged records to a CSV file. We will also log any errors that occur during this process.
Prerequisites
Before we begin, let's ensure that we have all the prerequisites in place:
1. Dynamics 365 CRM Instance: Access to a Dynamics 365 CRM instance is required, with the necessary privileges to perform merging operations on contact records.
2. Azure Active Directory (Azure AD) Application: Create an Azure AD application and note the clientId and clientSecret. These credentials will be used for authentication.
3. Development Environment: Use either Visual Studio or Visual Studio Code as your development environment to write and execute C# code.
4. NuGet Packages: Ensure that you have the following NuGet packages referenced in your project:
-
- Microsoft.CrmSdk.CoreAssemblies
- Microsoft.CrmSdk.XrmTooling.CoreAssembly
Creating the Console Application
Let's go through the steps to create the console application:
1. Create a New Console Application: Open Visual Studio or Visual Studio Code, create a new C# console application, and name it "ContactsMerge."
2. Add NuGet Packages: In your project, add references to the NuGet packages mentioned above if they are not already added.
3. Replace the Code: Replace the code in the Program.cs file with the provided code. The code includes the logic to connect to CRM, identify and merge duplicate contacts, and export the results to a CSV file.
Here's a detailed explanation of the key parts of the code:
Establishing CRM Connection
The CrmServiceClient class is used to establish a connection to the Dynamics 365 CRM instance. This class requires your Azure AD application's clientId and clientSecret for authentication.

Fetching Contacts
We use a QueryExpression to retrieve contact records from CRM. The query specifies the columns to retrieve and applies a filter only to fetch active contacts.

Merging Contacts
The application's core involves identifying and merging duplicate contacts based on criteria like full name, email, business phone, and mobile phone. This is done by constructing a FetchXML query and retrieving potential duplicate contacts.
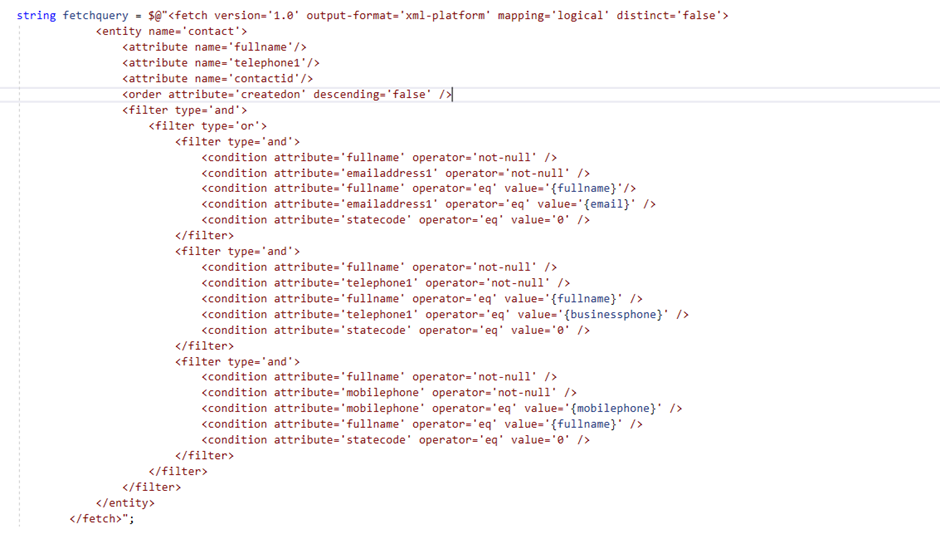
Merging Logic
The code then proceeds to merge the duplicate contacts. It selects the contact with the highest "thg_activitycount" (activity count) as the primary contact and merges the others into it using the MergeRequest and MergeResponse classes.
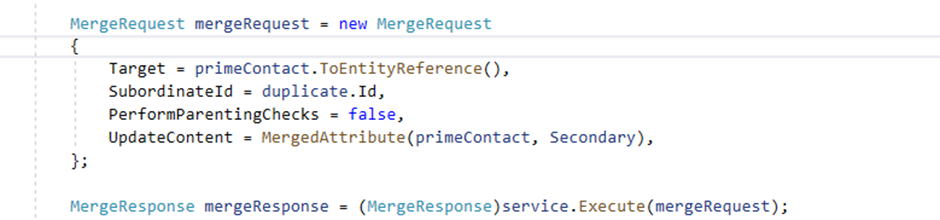
Exporting to CSV
The application constructs a CSV file with information about the merged records, including the primary contact ID, merged contact IDs, full name, email, business phone, and mobile phone. This CSV data is then written to a file.

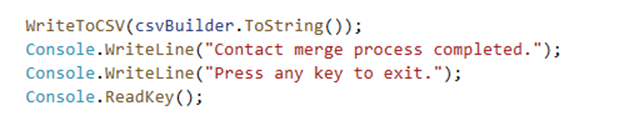
Conclusion
In this detailed guide, we've created a C# console application to merge contacts in Dynamics 365 CRM and export the results to a CSV file. The code includes error handling ensuring that issues are logged in the CSV file during the process. This tool can be valuable for data cleanup and consolidation within your CRM system.
You can customize and extend this application to meet your organization's requirements. Additional features like automation, scheduled runs, and integration with other systems can be implemented as needed. Happy coding!