What is Optimistic concurrency control?
The optimistic concurrency feature allows your applications to check if a table record has been altered on the server during the period between when your application initially fetched the record and when it attempts to modify or remove that record.
How to enable optimistic concurrency?
To activate the optimistic concurrency checking functionality while performing an UpdateRequest, you can achieve this by configuring the ConcurrencyBehavior property of the request as IfRowVersionMatches. Likewise, when executing a DeleteRequest, you would also adjust the ConcurrencyBehavior property accordingly.
When working within the .NET SDK context to make alterations to data, make sure to specify the ConcurrencyBehavior on the OrganizationServiceContext object. This value will be passed through to all the UpdateRequest and DeleteRequest messages used by the OrganizationServiceContext when SaveChanges () is called.
You can exclusively configure the optimistic concurrency behavior through an SDK API call. Currently, there is no option to adjust it within the web application interface.
How to apply optimistic concurrency using SDK for .NET?
You can specify the optimistic concurrency behavior for an operation by configuring the ConcurrencyBehavior property within the UpdateRequest or DeleteRequest classes.
The process of updating or deleting a row may be based on potentially outdated data. If the current data differs because it has been modified since retrieval, optimistic concurrency provides a mechanism to abort an update or delete operation. This allows you to retrieve the most recent data and determine whether to proceed accordingly.
To ascertain whether the row has been altered, there's no need to compare all the values individually. You can simply utilize the RowVersion property to check for changes.
The following example succeeds only when:
1. The RowVersion of the row in the database is 986323.
2. The account table is enabled for optimistic concurrency (EntityMetadata.IsOptimisticConcurrencyEnabled property is true)
3. The RowVersion property is configured for the row included in the request.
If the RowVersion does not match, an error message will be generated, stating: 'The version of the existing record does not match the provided RowVersion property.
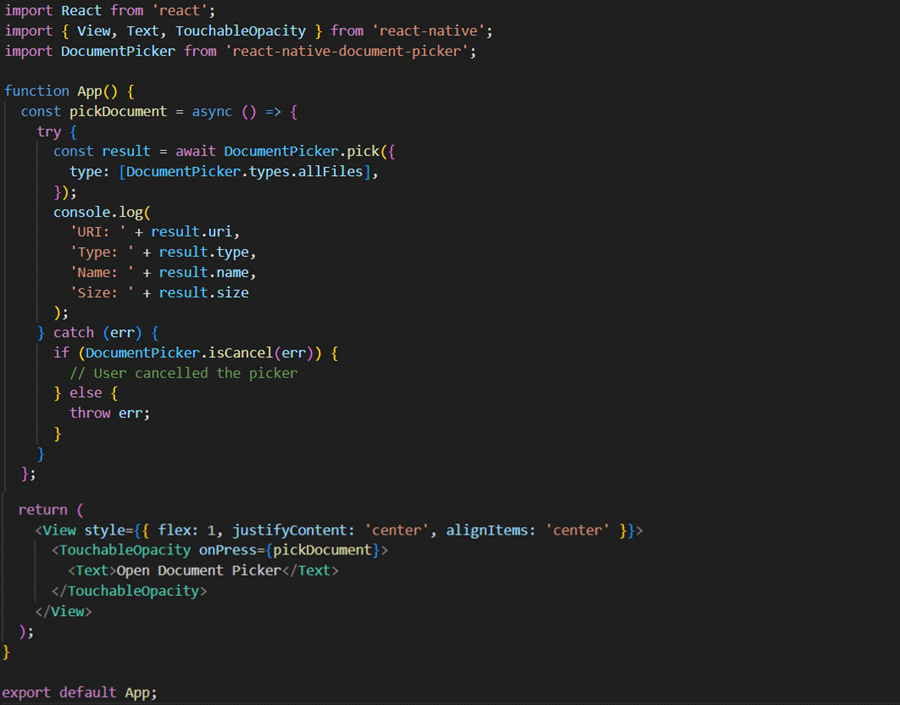
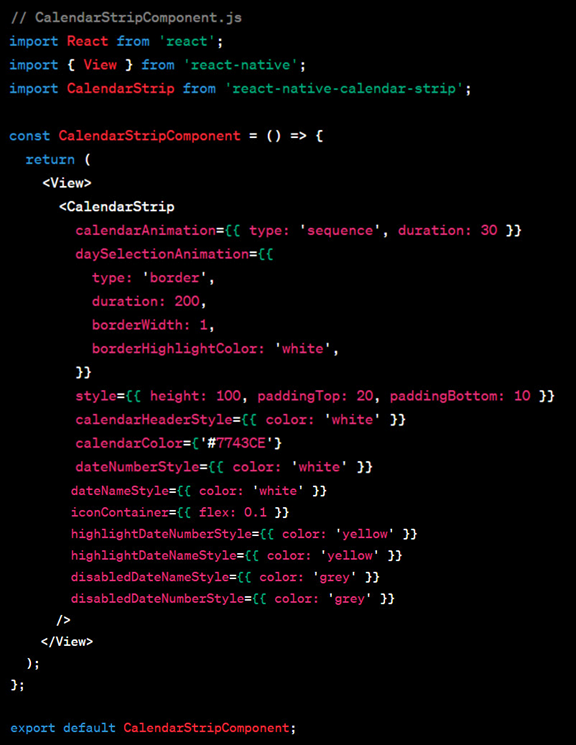
Preventing Simultaneous Modifications by Multiple Users on the same record-
The Dynamics CRM is used by multiple users. The likelihood of multiple users attempting to update the same record simultaneously is quite significant. In such a scenario, the modifications made by the most recent user will be preserved, leading to inconsistent data when users update the same fields simultaneously. Consequently, the Microsoft Xrm SDK offers a concurrency control mechanism when updating and deleting records using UpdateRequest and DeleteRequest, respectively.
Note: - To use the concurrency control mechanism of the Microsoft Xrm SDK, you must import the Microsoft.Xrm.Sdk namespace (i.e., using Microsoft.Xrm.Sdk;).
Here is a code implementation of a concurrency control mechanism:
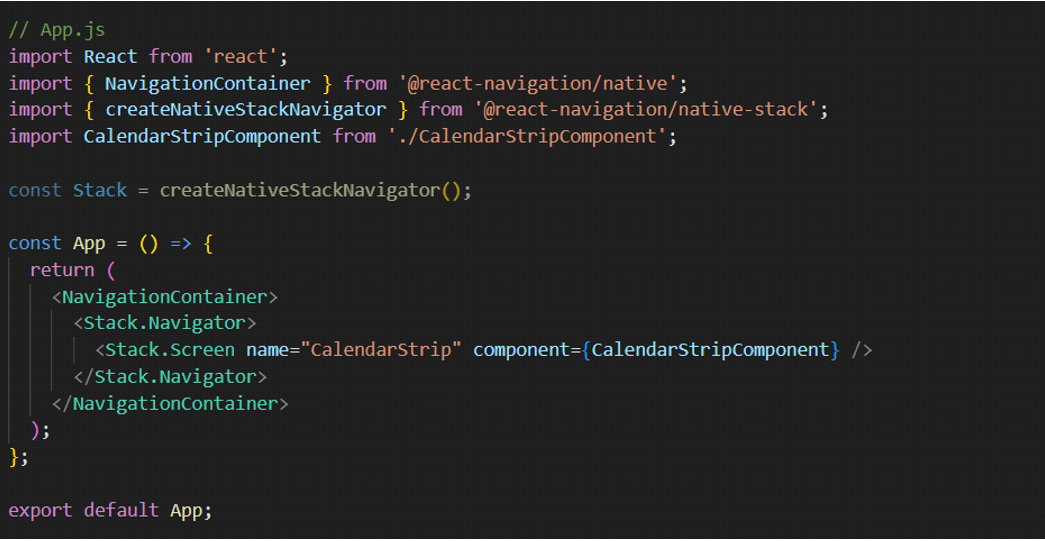
In that case, if you call service.Execute(), it will result in an exception: "The version of the existing record doesn't match the provided RowVersion property," and the execution will not be successful.
If you wish to perform the Update operation through code without any concurrency checks, simply modify this line as follows:
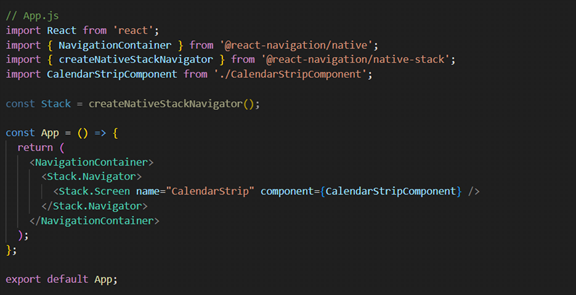
Similarly, you can maintain concurrency control when deleting the record using DeleteRequest, as outlined below:
