In my previous post here I had discussed about convention based routing which is the default way in Web API. Check it out here.Starting with Web API 2 Microsoft introduced attribute based routing. Which is way more simpler. The reason for introducing this new feature was two folds, one it is easier to understand and maintain, second is the kind of REST calls required.
Let me elucidate it with an example
GET /profile/20011/ContactNumber
GET /profile/2011/EmailAddress
Not to say that this cannot be achieved by traditional methods but it is much harder to understand.
So Microsoft came up with Attribute Routing.
Let us take an example
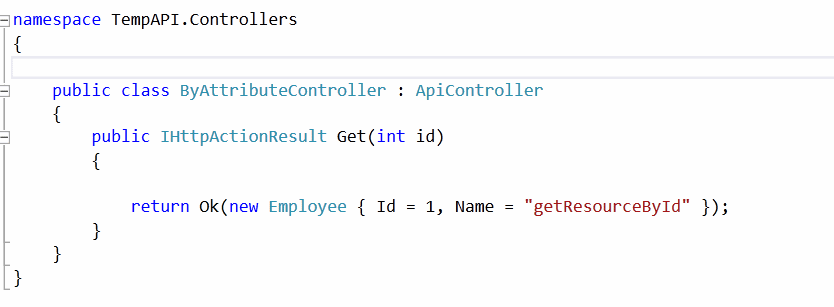
So for our first example let us assume that you want the route to read some thing like this
www.mysidye.com/Employee
In this case we use a prefix called [Route(“Employee”)] before the action needed.
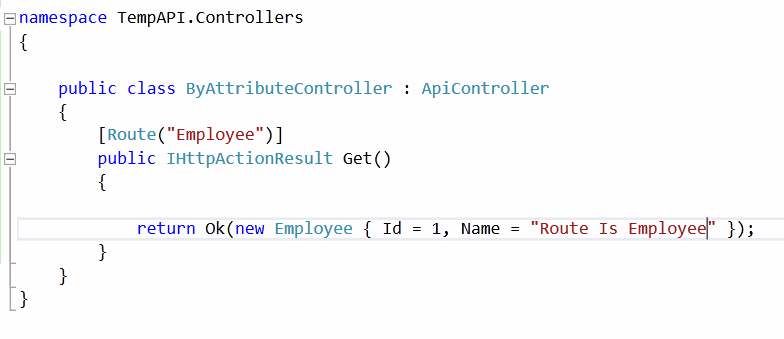
Parameter’s in End Point
Pretty simple isn’t it? Now let’s say you want the endpoint to be something like this, where 1 is the Id of the Employee, for whom you need the profile.
www.mysidye.com/Profile/56
Using Route Prefix this becomes pretty simple
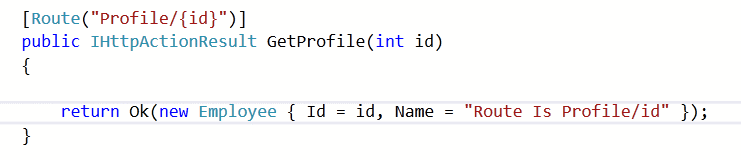
Now let it take a step further and this time we need all the orders for a particular employee with id 501
www.mysidye.com/Profile/56/Orders
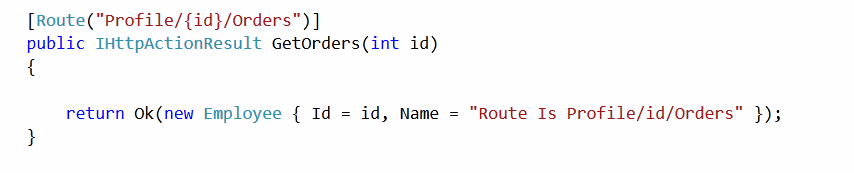
As you can see each parameter is written with curly braces and the function names the parameter exactly the same.
So does that mean we can use only a single parameter? No!
Let’s take another example, I want the order no 345 for the Employee profile with id of 56
www.mysidye.com/Profile/56/Order/345

Parameter Type
If we want to pass some parameter which has a type different from number, can we do it? Yes sure, let me modify the earlier example and get the profile based on name instead of id(not a good idea). Assumption is that name will always be unique and never repeated
www.mysidye.com/Profile/SudeepMukherjee/Order/345

As you can see I have decorated the parameter with the type. It looks fine but there is slight issue with this type, it only accepts alphabets and will fail if you pass a blank space. Please find the table below as a guide for your reference.
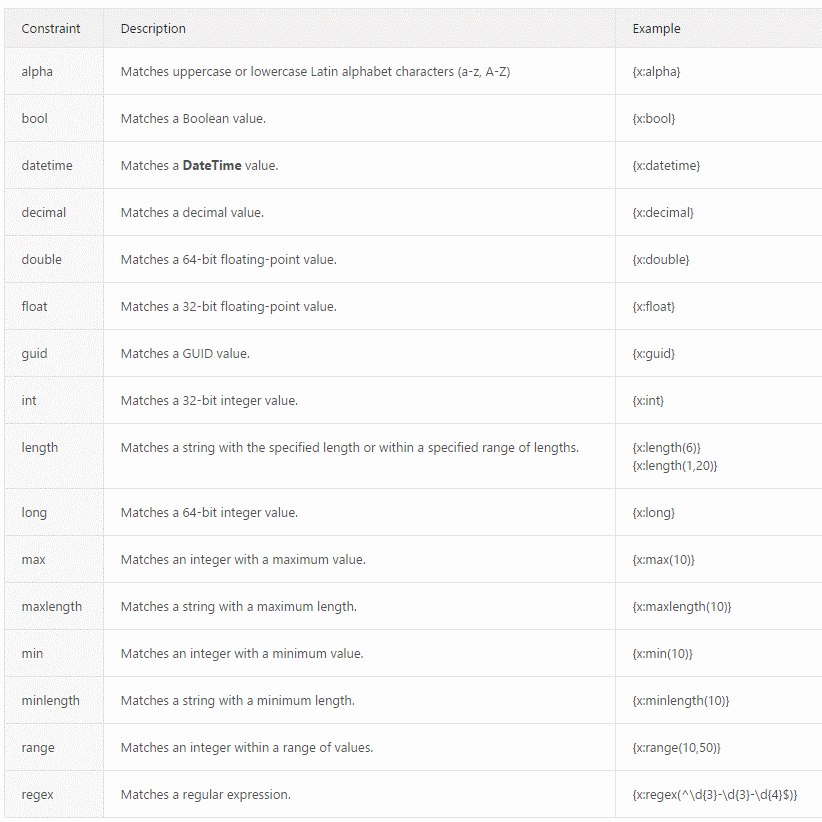
It is pretty much impossible to discuss all the options in one article but you can find it out by yourself how to use all of them.
RoutePrefix
Till now we have concentrated on defining the Rest end point based on the action. In our simple scenario it is pretty easy but in real world this is seldom the case. Your project will have multiple controllers and most probably some of them will have over lapping action names, even after you changing the route string.
In such a case we can put the RoutePrefix on top of our class/controller. Continuing from the earlier example let us assume we need the controller name to be part of the Rest endpoint. (Master is the name given to our class)
www.mysidye.com/Master/Profile/SudeepMukherjee/Order/345
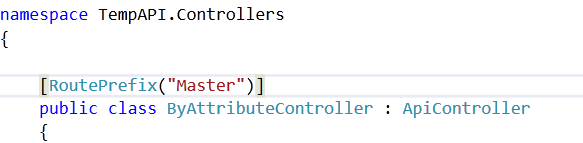
HTTP Methods
All the examples shown till now have been simple Get request but WebAPI2 is not restricted to only one verb. Following verbs are available to you for your usage.
· [HttpDelete]
· [HttpGet]
· [HttpHead]
· [HttpOptions]
· [HttpPatch]
· [HttpPost]
· [HttpPut]
Let’s assume that you are not following the conventions and the action name does not contain the Http verb, so how do you make Web API understand the verb you are using. Simple!
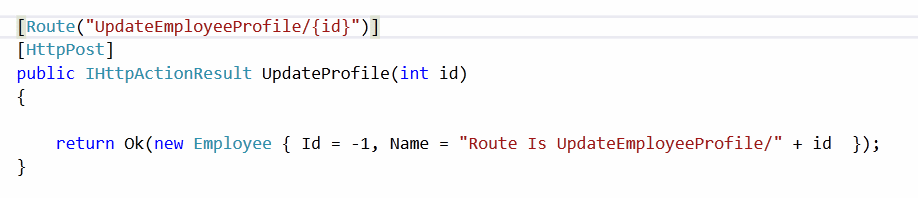
As you can see I have adorned the top of the action with the verb [HttpPost], this will make the Web API understand this is not a get request but a post request.
I hope I have made a few points clear to you and made the confusing routing a little easy, in my next article I will post a simple JavaScript code to call these webapi’s .